Introduction
Working with images in Python gives you a range of development capabilities, from downloading image with an URL to retrieving photo attributes. But, how exactly can you get access to these pictures in the first place?
In this article, you'll walk through several different methods used to download images in Python.
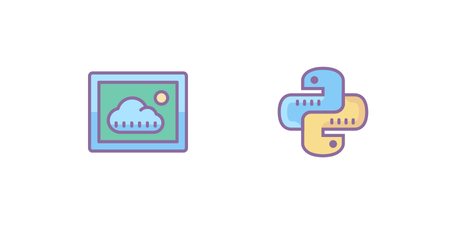
Prerequisites
To fully benefit from this post, you should have the following:
β Some experience with Python 2 or Python 3 π.
β Python 3 installed on your local machine. There is one script compatible with Python 2 in the Urllib Package section.
Using Python to download and save an image from URL
Β
Using the Requests Package
Being the most popular HTTP client in Python, the Requests package is elegant and easy to use for beginners. Many developers consider it a convenient method for downloading any file type in Python.
Assuming you have Python 3 installed to your local environment, create a directory mkdir download-images-python
and add in a requests_python_img_dl.py
. Once that file is opened, install and import the following packages:
import requests # request img from web
import shutil # save img locally
Once you've imported those files, create a url
variable that is set to an input
statement asking for the image URL.
url = input('Please enter an image URL (string):') #prompt user for img url
Additionally, create another variable also set to an input statement, file_name
:
file_name = input('Save image as (string):') #prompt user for file name
In the next line of code, implement the get()
method from the requests module to retrieve the image. The method will take in two parameters, the url
variable you created earlier, and stream: True
by adding this second argument in guarantees no interruptions will occur when the method is running.
res = requests.get(url, stream = True)
The copyfileobj()
method to write your image as the file name, builds the file locally in binary-write mode and saves it locally with shutil
. Although it's not necessary, it's good to check if the image was retrieved successfully using Requestβs Status Code in a conditional statement.
if res.status_code == 200:
with open(file_name,'wb') as f:
shutil.copyfileobj(res.raw, f)
print('Image sucessfully Downloaded: ',file_name)
else:
print('Image Couldn\'t be retrieved')
Your finished script should look something like this:
import requests # request img from web
import shutil # save img locally
url = input('Please enter an image URL (string):') #prompt user for img url
file_name = input('Save image as (string):') #prompt user for file_name
res = requests.get(url, stream = True)
if res.status_code == 200:
with open(file_name,'wb') as f:
shutil.copyfileobj(res.raw, f)
print('Image sucessfully Downloaded: ',file_name)
else:
print('Image Couldn\'t be retrieved')
Execute your script by running the following command in your terminal:
python requests_python_img_dl.py
Your downloaded images will save to the newly created download-images-python
directory. Congratulations, you can now request all the image downloads your heart desires π.
Downloading Images Using urllib
Another favored method for downloading data in Python is through urllib
, a package that collects several modules for working with URLs, including:
-
urllib.request for opening and reading.
-
urllib.parse for parsing URLs.
-
urllib.error for any exceptions raised by urllib.request.
-
urllib.robotparser for parsing robot.txt files.
To learn more about the urllib
module, refer back to the documentation
here
, but now that you know the basics its time to get started!
If urllib
is not present in your current environment, install it by executing the code below:
pip install urllib
Note that if you are using Python 2, unfortunately, this code will not work with your environment, but the end of this section includes a Python 2 compatible script.
Once you have urllib
installed, create a new directory for your project, mkdir python-image-downloads
. This step does not need to be repeated if you already created it in the previous section. Within that directory create an images folder, as well as a dl_img.py
file. Navigate to your dl_img.py
file, and at the top insert the following line of code to import the urllib
package:
import urllib.request
With your module imported, your task is to make a user-friendly script that will allow you to download images quickly and organized. First, create a url
variable from an input function:
url = input('Please enter image URL (string):')
Then decide what you would like your image file to save as, using another input statement:
file_name = input('Save image as (string):')
With these two variables that hold the data needed to download and organize your newly created image files, write the code that saves the image(s). Begin by defining a function that takes in three parameters, your url
variable, the designated file_path
you would like to save the image to, and the file_name
set previously.
def download_image(url, file_path, file_name):
Inside the function create a full_path
to where the image is going to be saved to. The image's full path will be the file_path
with the file_name
concatenated, and a '.jpeg'
string added on to the end. *Similarly if you wanted to save your image as a PNG you would use '.png'
.
def download_image(url, file_path, file_name):
full_path = file_path + file_name + '.jpg'
To create the code that actually downloads the picture, you'll need to integrate the urllib.request
and urlretrieve
which will automatically download and save the image based on the arguments given, β¨thankfully you just made them!β¨
def download_image(url, file_path, file_name):
full_path = file_path + file_name + '.jpg'
urllib.urlretrieve(url, full_path)
Call that function at the end of your script that should like similar to this:
import urllib.request
def download_image(url, file_path, file_name):
full_path = file_path + file_name + '.jpg'
urllib.urlretrieve(url, full_path)
url = input('Please enter image URL (string):')
file_name = input('Save image as (string):')
download_image(url, 'images/', file_name)
When you call download_image
you'll need to pass through three arguments again, this time it will be your url
, the file path which is the 'images/'
folder you created in the beginning, and the file_name you chose.
Believe it or not, that's it! As long as you're in your python-image-downloads
directory, run your script in your terminal with the code below:
python dl_img.py
Great job! You just downloaded your first image in Python using the urllib
package π.
βοΈ If you have not yet upgraded Python 3 you may find yourself receiving several errors regarding urllib.request
, the following code should be compatible with Python 2.
import urllib2
def download_image(url):
request = urllib2.Request(url)
img = urllib2.urlopen(request).read()
with open (file_name + '.jpg', 'w') as f: f.write(img)
url = input('Please enter image URL (string):')
file_name = input('Please enter file name (string):')
download_image(url)
*Unlike Python 3, version 2 does not support the urllib.request
package. As an alternative you can import
urlopen(request).read()
to read the image url, and then download it to your local environment. However, the image will now be saved directly to the python-image-downloads
directory instead of the images
folder.
Using Wget Module
In addition to the Requests
and Urllib
packages, it's also possible to download images in Python by employing the
wget
module. If you have already made your python-image-download
navigate inside, if not create it now. Within the directory create a wget_img_dl.py
file and import the wget
module like so:
import wget
Once you have wget installed and imported, set a url
variable equal to an input statement that assigns an image address:
url = input('Please enter image URL (string):')
Utilizing wget.download
pass in the url
variable as an argument, and set it equal to a file_name
variable you'll be able to access it by afterwards.
file_name = wget.download(url)
print('Image Successfully Downloaded: ', file_name)
The full script should like close to this:
import wget
url = input('Please enter image URL (string):')
file_name = wget.download(url)
print('Image Successfully Downloaded: ', file_name)
That's all! You can run your script π:
python wget_img_dl.py
We also wrote an article about using Python with wget , it's another great way of using wget with Python.
π‘ Love web scraping in Python? Check out our expert list of the Best Python web scraping libraries.
Conclusion
As always, each of these methods carries pros and cons. If you have problems installing the Requests package or want fewer dependencies in your program urllib might be your best option. However, the Requests module has become a popular and reliable way of downloading anything in Python, with even the urllib documentation recommending it as high-level HTTP client interface.
If you're looking for even more ways to download images and file types from the web with Python, I encourage you to check out the post, The best Python HTTP clients for 2021 .
I hope you found this post enjoyable. You can also access the source code here . Happy Scraping! π