APIs for dummies: What is an API and how do APIs work?
Ever wondered how your favorite apps and websites effortlessly share information with each other? That's where APIs come in! API stands for Application Programming Interface, but don't let that technical-sounding name scare you. Simply put, APIs are like bridges that allow different software systems to communicate and share data or features with each other.
Think about it this way: when you use a weather app on your phone, that app doesn't generate the weather data itself—it fetches it from another service using an API. Or, when you log into a new website using your Google account instead of creating yet another username and password, that's thanks to an API that connects the website to Google's system to verify your info.
APIs are the unsung heroes of the digital world, making it easier for developers to build complex applications without reinventing the wheel every time. In this article, we'll cover various API types and break them down in simple terms.
Here's some of what we'll cover in this APIs for dummies guide:
What is an API?
APIs, APIs everywhere!
What is a REST API?
What is an OS API?
What are database APIs?
Frequently asked questions
Conclusion
What is an API? Can you explain it in super-simple terms?
Sure! Let's break it down with a little story. Imagine John heads to a bar on the weekend to grab a couple of beers. Does John go straight to the beer keg and pour himself a drink? Of course not! Instead, he asks the bartender, Bob, to pour him a Guinness. (Personally, I'm more into English ciders or some Latvian brews.) Bob picks the right glass, pours the drink, and hands it over to John. Believe it or not, that's basically how APIs work.
In this scenario, John is like a client making a request to Bob, the bartender (who's the API), to get a drink. If John is with his friend Ann, she might ask Bob for some soda and buffalo wings. Bob, being the same API, handles her request too. But when it comes to the wings, Bob probably gets help from the kitchen (the back-end). The cool part is, John and Ann don't need to know how the drinks are poured or how the wings are cooked—they just get what they asked for and can enjoy their evening.
It's all about simplicity
This example shows that APIs let different clients—like web or mobile apps—ask a third-party service to do something for them. It could be something simple, like returning certain data, or more complex, like storing information or sending an email. The key takeaway? For the client, solving the task is as easy as sending a request to the right API.
The client doesn't need to understand all the complexities behind the system they're using. And they don't get direct access to the system—just like John and Ann don't go behind the bar. Instead, they rely on an intermediary to carry out the task.
APIs, APIs everywhere!
What can we achieve with APIs?
APIs open up a world of possibilities, making it easier to build powerful and flexible applications. Here are a few simple examples:
Accessing data from anywhere: Want to display live weather updates on your website? APIs can connect your site to a weather service that provides real-time data. You don't have to build a weather station—just use the API!
Simplifying user logins: Tired of creating new usernames and passwords for every app or site? APIs let you log in with your existing accounts, like Google or Facebook, making the process faster and more secure.
Interacting with your operating system: APIs aren't just for web apps! For example, when a software program interacts with your computer's operating system to save a file or manage memory, it uses the OS's API. This allows different software to work smoothly with your computer's hardware and other software without needing to understand the inner workings of the OS.
These are just a few examples, but APIs can do so much more. They allow apps to talk to each other, automate tasks, and bring together different services into one seamless experience.
Types of APIs
APIs are incredibly useful and have become so widespread that they can be found everywhere in the world of information technology. Let's explore some of the most common types of APIs you might encounter:
REST (Representational State Transfer) API: This is the most popular type of API used in web services today. REST APIs rely on standard HTTP methods like GET, POST, PUT, and DELETE to allow communication between clients (like your web browser) and servers.
SOAP (Simple Object Access Protocol) API: SOAP is an older protocol compared to REST, but it's still used in many enterprise environments. It's more rigid and standardized, relying exclusively on XML for message formatting.
GraphQL API: Developed by Facebook, GraphQL is a more modern API that gives clients much more control over the data they receive. Instead of getting all data from a server endpoint, clients can specify exactly what they need, reducing the amount of data transferred and improving efficiency.
WebSocket API: Unlike REST, which operates on a request-response basis, WebSocket APIs enable real-time, two-way communication between a client and a server. This is ideal for applications that need to send and receive data continuously, like chat apps or online gaming.
Operating System APIs: These APIs are provided by operating systems to let software applications interact with the underlying hardware and other software components. For example, Windows API, POSIX API (used in UNIX-based systems), and macOS API allow applications to perform tasks like file management, process control, and network communication.
Database APIs: These are specialized APIs designed for interacting with databases. They allow applications to perform operations like querying, updating, and managing data stored in a database. Examples include SQL-based APIs like ODBC (Open Database Connectivity) and JDBC (Java Database Connectivity), as well as APIs for NoSQL databases like MongoDB's API.
Hardware APIs: These APIs provide a way for software to communicate with hardware components. For example, DirectX and OpenGL are APIs that allow software to interact with graphics hardware to render images and animations. Similarly, manufacturers of sensors like accelerometers or cameras often provide APIs that allow developers to access and use data from these devices in their applications.
These are just some examples of the different types of APIs out there, each designed to solve specific problems and work in various environments. Next, we'll dive deeper into certain API types that you might encounter.
What is a REST API?
A REST API (Representational State Transfer) is one of the most common ways for web services to communicate with each other. It's a type of API that allows different systems to interact using HTTP, the same protocol your web browser uses to load websites. REST APIs are designed to be simple and stateless, meaning each request from a client contains all the information the server needs to process it—there's no need for the server to remember anything from previous interactions.
Verbs and endpoints: Ordering from the menu
Let's bring back our bar analogy to make things clearer. Imagine you're at a bar, and the menu lists everything you can order—drinks, snacks, etc. In the world of REST APIs, this menu represents the endpoints. Each item on the menu is like a different endpoint you can interact with.
Now, let's talk about how you actually interact with these endpoints. This is where the HTTP verbs come in, which are like the specific actions you ask the bartender to perform:
GET: This is like asking the bartender for information. For example, you might get the menu, get the bill, or get details about the soup of the day. In REST API terms, GET is used to retrieve data from the server. For instance, you might use a GET request to ask for a list of available products.
POST: When you're ready to order, you post your request. This could be ordering wings or asking the bartender to pour you a drink. In REST APIs, POST is used to create something new on the server. For example, you might use POST to submit a new order or add an item to your shopping cart.
PUT: Imagine you ordered a beer, but then you decide you want a cider instead. Or maybe you want to add more items to your existing order. You'd ask the bartender to update your order. PUT is used to update existing data on the server, like updating your profile information on a website.
DELETE: Maybe you've decided you no longer want those wings you ordered, so you ask the bartender to cancel it. DELETE is used to remove data from the server, like deleting an unwanted post from your social media account.
These are the most common verbs, but there are others as well.
Endpoints: Where you make your requests
So, where exactly do you make these requests? That's where endpoints come in. An endpoint is a specific URL that corresponds to a particular part of the service, just like how a menu item corresponds to a specific dish or drink.
For example:
/menu
might be an endpoint that lets you GET a list of available drinks and snacks. You can even customize this request using GET parameters, like/menu?filter=snack,burgers
, to show only snacks and burgers. In this case,filter
is the GET parameter, and the values come after the equal sign. These parameters can also be used with other HTTP verbs./orders
might be where you POST a new order for wings or a drink./orders/123
could be used to PUT updated details for order number 123./orders/123
could also be used to DELETE that specific order.
These endpoints guide the REST API on what you want to interact with, much like how you'd choose a specific item from the menu.
The anatomy of a REST API request
Now that we've covered the basics of verbs and endpoints, let's get a bit more technical and break down what a typical REST API request actually contains. Whenever you make a request to a REST API, there are a few key parts involved:
Verb (or Method): We've already talked about this—GET, POST, PUT, DELETE. This tells the server what action you want to perform.
Endpoint: This is the specific URL that points to the resource you're interacting with, like
/menu
to see what's available or/orders/123
to update an order. Think of this as telling the bartender exactly what you want from the menu.Headers: This is where things get a bit more customized. Headers are like the extra instructions you give to the bartender when placing your order. Here are a couple of important headers:
Content-Type: This header tells the server what kind of data you're sending with your request. For example, if you're sending JSON data, you'd set the
Content-Type
toapplication/json
. It's like telling the bartender how you're placing your order—whether it's written down or spoken aloud.Accept: This header tells the server what kind of response you expect back. For example, you might want the server to return data in JSON format (
application/json
). It's like telling the bartender how you'd like your order served—maybe you want your drinks extra cold, or your food extra spicy. TheAccept
header customizes how you want the response to be delivered.Authorization: Although we'll get into this more later, the Authorization header is often used to prove who you are when making a request. It's like showing your ID to prove you're of legal drinking age before placing an order at the bar.
Body: Not every request needs a body, but when you're creating or updating something (like with POST or PUT), the body contains the actual data you're sending to the server. In our bar analogy, this is the detailed information about your order—what you want to eat or drink, how much of it, any special instructions, etc. For example, if you're ordering wings, the body might include details like “12 buffalo wings with extra sauce.”
The anatomy of a REST API response
After you've made a request to a REST API, the server sends back a response. Just like when you place an order at a bar, the bartender (server) will respond in some way—whether it's handing over your drink, giving you a bill, or letting you know if something went wrong. Let's break down what this response typically contains:
Status Code: This is like the bartender giving you a thumbs up or a polite apology. The status code tells you how the server handled your request:
200 OK: Everything went smoothly! Your order was successful. This is like the bartender smiling and handing you your drink.
201 Created: Your order was successfully placed, and something new was created (like a new order in the system).
400 Bad Request: Uh-oh, something went wrong with your request. Maybe you asked for something that's not on the menu. The bartender might say, “Sorry, we don't serve that here.”
404 Not Found: The server couldn't find what you were asking for—like if you tried to order a drink that doesn't exist. The bartender might respond with, “Sorry, we don't have that drink available.”
500 Internal Server Error: Something went wrong on the server's side. Maybe the bartender dropped your drink before it got to you. In tech terms, this means there was an unexpected problem on the server.
Headers: Just like the headers you send in a request, the server's response comes with headers too. These are extra details that the bartender gives you along with your order:
Content-Type: This header tells you what format the response data is in, such as
application/json
. It's like the bartender handing you a cocktail with a note that says what's in it.Content-Length: This tells you the size of the response. It's like the bartender mentioning whether your drink is a small, medium, or large.
Body: If everything went well and the server has some data to send back, it will be in the body of the response. This is the actual content you were waiting for—like the drink or food you ordered. For example, if you made a GET request to
/menu
, the body might contain a list of all the available drinks and snacks in JSON format. If you POSTed a new order, the body might return a confirmation with details of what you ordered.
What is an API key/token?
An API key is like your personal ID when interacting with a server. It's a long string of random letters and numbers that acts as a password for your API requests. This key can be sent as part of the URL (like a GET parameter) or, more securely, placed in the Authorization header we talked about earlier. Be careful to not share your API keys as they are like your password.
Why is an API token needed?
Think of an API token as your way of proving who you are to the server. In many cases, the server needs to know exactly who is making a request, what permissions they have, what they're allowed to do, and what actions are off-limits. For instance, some actions might only be available to users with certain privileges, like admins. Also, many APIs have usage limits or subscription plans, so to prevent overuse or abuse, the server needs to identify each user.
Now, here's where the analogy comes in. Let's say you're at a bar, and after you've enjoyed your meal and drinks, it's time to pay. The bartender needs to keep track of what you've ordered throughout the evening, right? So, they assign you a “token,” which might just be the number of the table where you're sitting. In some places, you might even get a literal token or card with a number on it when you place your order. This token helps the bartender remember your tab—how much you've ordered and what you still owe.
In the same way, an API token helps the server remember who you are and what actions you're allowed to take. It ensures that your requests are handled correctly based on your permissions.
Keeping your API token safe
Just like you wouldn't want to lose a card that tracks your bar tab (you'd still have to pay for everything, after all!), it's crucial to keep your API token safe. If someone else gets hold of it, they could make requests to the server as if they were you—potentially causing all sorts of trouble.
Example: Using ScrapingBee API
Let's put what we've learned into practice. We'll use the ScrapingBee REST API to scrape data from any website without getting blocked. With this API, you can easily set up a premium proxy, manage cookies, take screenshots of web pages, and much more.
To get started, sign up for a free ScrapingBee trial; no credit card is required, and you'll receive 1,000 credits to begin. Each request typically costs around 25 credits.
Once you've signed up, log in to your dashboard and copy your API token—you'll need this to send requests.
Next, install the ScrapingBee Python client by running:
pip install scrapingbee
Now, you're ready to start scraping with the following Python snippet:
from scrapingbee import ScrapingBeeClient
client = ScrapingBeeClient(api_key="YOUR_API_KEY")
response = client.get(
YOUR_URL_HERE,
params={
'premium_proxy': True, # Use premium proxies for tough sites
'country_code': 'gb',
"block_resources": True, # Block images and CSS to speed up loading
'device': 'desktop',
"wait": "1500", # Milliseconds to wait before capturing data
'js_scenario': {
"instructions": [ # Automate interactions with the webpage
{"wait_for": "#slow_button"},
{"click": "#slow_button"},
{"scroll_x": 1000},
{"wait": 1000},
{"scroll_x": 1000},
{"wait": 1000},
]
},
headers={"key": "value"}, # Custom headers
cookies={"name": "value"} # Custom cookies
# Optional screenshot settings:
# "screenshot": True,
# "screenshot_full_page": True,
}
)
print(response.content)
For more tips and best practices, check out our guide on Scraping without getting blocked.
How to create a simple REST API with Python and Flask
Creating your own API is easier than you might think, especially with Python and Flask. Flask is a lightweight web framework that lets you build APIs quickly and easily. Let's walk through how to set up a simple API with just a couple of endpoints.
Set up your environment
First, make sure you have Python installed. Then, install Flask by running:
pip install flask
Create a REST API for a bar
Create a new Python file (let's call it app.py
) and set up a basic Flask app:
from flask import Flask, jsonify, request
app = Flask(__name__)
# Sample data: Menu items (beers)
menu = [
{"id": 1, "name": "Lager", "price": 5.0},
{"id": 2, "name": "IPA", "price": 6.5},
{"id": 3, "name": "Stout", "price": 7.0},
]
# Sample data: Orders (initially empty)
orders = []
# Home endpoint
@app.route('/')
def home():
return "Welcome to our bar!"
# GET endpoint to retrieve the menu
@app.route('/menu', methods=['GET'])
def get_menu():
return jsonify(menu)
# POST endpoint to place an order
@app.route('/order', methods=['POST'])
def place_order():
new_order = request.json
new_order_id = len(orders) + 1
new_order['id'] = new_order_id
orders.append(new_order)
return jsonify({"order_id": new_order_id}), 201
# GET endpoint to retrieve the bill for a specific order
@app.route('/bill/<int:order_id>', methods=['GET'])
def get_bill(order_id):
order = next((order for order in orders if order["id"] == order_id), None)
if order:
total_price = order['price']
return jsonify({"order_id": order_id, "total_price": total_price})
else:
return jsonify({"error": "Order not found"}), 404
if __name__ == '__main__':
app.run(debug=True)
Save the file and run it with:
python app.py
Your Flask API should now be running locally on http://127.0.0.1:5000/.
Test your endpoints
Let's do some testing with cURL command line tool.
First, run:
curl http://127.0.0.1:5000
You should see a welcoming message printed to the terminal. This is a basic GET request sent to the server root (/
).
Now, let's view the menu:
curl http://127.0.0.1:5000/menu
The server will respond with:
[
{"id": 1, "name": "Lager", "price": 5.0},
{"id": 2, "name": "IPA", "price": 6.5},
{"id": 3, "name": "Stout", "price": 7.0}
]
Nice, let's place an order:
curl -X POST http://127.0.0.1:5000/order -H "Content-Type: application/json" -d "{\"name\": \"IPA\", \"price\": 6.5}"
You'll get your order id:
{
"order_id": 1
}
When it's time to pay, you can ask for the check:
curl http://127.0.0.1:5000/bill/1
And here's your total:
{
"order_id": 1,
"total_price": 6.5
}
And that's it! You've created a simple REST API that simulates a bar where you can view a menu, place orders, and get the total bill for a specific order.
What is an OS API?
An OS API (Operating System API) allows software applications to interact with the underlying operating system's features and hardware. This type of API provides a way for programs to perform tasks like file management, process control, and accessing system resources (e.g., memory, network, or peripheral devices) without needing to dive into the complexities of how the operating system works internally.
Example: Using the Windows API with Python
One common example of an OS API is the Windows API (also known as WinAPI), which provides a set of functions for interacting with the Windows operating system. Using Python, you can access some of these functions via the ctypes
or pywin32
libraries.
Here's a simple example that shows you how to take a screenshot and play a system sound using Python:
import ctypes
from PIL import ImageGrab
import os
# Function to play a system sound (Beep)
def play_sound():
# Beep with frequency 1000 Hz and duration 500 ms
ctypes.windll.user32.MessageBeep(0xFFFFFFFF)
# Function to take a screenshot
def take_screenshot(filename="screenshot.png"):
# Capture the screen
screenshot = ImageGrab.grab()
# Save the screenshot to the specified file
screenshot.save(filename)
# Play the system sound
play_sound()
# Take a screenshot
screenshot_filename = "screenshot.png"
take_screenshot(screenshot_filename)
print(f"Screenshot saved as {screenshot_filename}.")
Explanation:
ctypes.windll.user32.MessageBeep(0xFFFFFFFF)
plays a simple beep sound. The parameter0xFFFFFFFF
plays the default system sound.ImageGrab.grab()
captures the current screen using OS API.screenshot.save(filename)
saves the captured screenshot as a file, with the default filename being screenshot.png.
Make sure to install Pillow before running the script:
pip install pillow
When you run this script, your system will play a beep sound, and a screenshot will be taken and saved as screenshot.png
in the current working directory.
What are database APIs?
A database API (Application Programming Interface) allows applications to interact with a database management system (DBMS). Through a database API, you can perform operations such as querying, updating, inserting, and deleting data in a database. These APIs provide a standardized way to communicate with different types of databases, whether they are SQL-based (like MySQL or PostgreSQL) or NoSQL-based (like MongoDB).
SQL-based APIs
SQL-based database APIs, such as ODBC (Open Database Connectivity) or JDBC (Java Database Connectivity), enable applications to execute SQL queries and retrieve results from relational databases. These APIs translate high-level SQL commands into low-level instructions that the DBMS can process.
NoSQL-based APIs
NoSQL databases, like MongoDB, often provide their own APIs that are designed to interact with their non-relational data models. These APIs typically allow for operations like inserting documents, querying data based on specific criteria, and updating records.
Example: Using a database API in Python
For example, in Python, the sqlite3
module provides an API to interact with SQLite databases. Here's a simple example:
import sqlite3
# Connect to a SQLite database (or create it if it doesn't exist)
conn = sqlite3.connect('example.db')
# Create a cursor object to interact with the database
cursor = conn.cursor()
# Create a table
cursor.execute('''CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)''')
# Insert a new record
cursor.execute('''INSERT INTO users (name) VALUES ('Alice')''')
# Commit the changes
conn.commit()
# Query the database to retrieve data
cursor.execute('''SELECT * FROM users''')
rows = cursor.fetchall()
# Print the retrieved data
for row in rows:
print(f"ID: {row[0]}, Name: {row[1]}")
# Close the connection
conn.close()
If you run the script, it will output something like:
ID: 1, Name: Alice
This shows that the record was successfully inserted into the database and then retrieved and printed.
Frequently asked questions
What is an API?
An API (Application Programming Interface) is a set of rules that allows different software applications to communicate with each other. It defines how requests and responses should be formatted and processed, enabling seamless interaction between systems.
What is an API integration?
API integration is the process of connecting two or more software applications via their APIs, allowing them to exchange data and perform tasks together. This integration enables different systems to work in sync, automating processes and enhancing functionality.
What is a REST API?
A REST API (Representational State Transfer) is a type of API that uses standard HTTP methods like GET, POST, PUT, and DELETE to allow communication between clients and servers. It is stateless, meaning each request is independent, and it typically returns data in formats like JSON or XML.
What is an API key?
An API key is a unique identifier used to authenticate a user or application when making API requests. It acts like a password, ensuring that only authorized users can access the API and its services.
🤖 Become a Web Scraping Ninja with our guide on What is Web Scraping and How to Scrape Any Website which includes quick start code to get you mining the internet for data in no time.
Conclusion
APIs are the backbone of modern software development, enabling different applications and systems to communicate and work together seamlessly. Whether you're working with web services, operating systems, or databases, understanding how to interact with APIs is an essential skill for any developer.
As you continue your journey in software development, keep experimenting with APIs, exploring new libraries, and building more complex integrations. The possibilities are endless, and APIs will be your key to unlocking them.
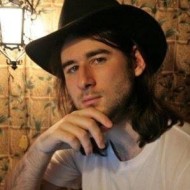
Ilya is an IT tutor and author, web developer, and ex-Microsoft/Cisco specialist. His primary programming languages are Ruby, JavaScript, Python, and Elixir. He enjoys coding, teaching people and learning new things. In his free time he writes educational posts, participates in OpenSource projects, tweets, goes in for sports and plays music.