Data parsing is the process of taking data in one format and transforming it to another format. You'll find parsers used everywhere. They are commonly used in compilers when we need to parse computer code and generate machine code.
This happens all the time when developers write code that gets run on hardware. Parsers are also present in SQL engines. SQL engines parse a SQL query, execute it, and return the results.
In the case of web scraping, this usually happens after data has been extracted from a web page via web scraping. Once you've scraped data from the web, the next step is making it more readable and better for analysis so that your team can use the results effectively.
A good data parser isn't constrained to particular formats. You should be able to input any data type and output a different data type. This could mean transforming raw HTML into a JSON object or they might take data scraped from JavaScript rendered pages and change that into a comprehensive CSV file.
Parsers are heavily used in web scraping because the raw HTML we receive isn't easy to make sense of. We need the data changed into a format that's interpretable by a person. That might mean generating reports from HTML strings or creating tables to show the most relevant information.
Even though there are multiple uses for parsers, the focus of this blog post will be about data parsing for web scraping because it's an online activity that thousands of people handle every day.
How to build a data parser
Regardless of what type of data parser you choose, a good parser will figure out what information from an HTML string is useful and based on pre-defined rules. There are usually two steps to the parsing process, lexical analysis and syntactic analysis.
Lexical analysis is the first step in data parsing. It basically creates tokens from a sequence of characters that come into the parser as a string of unstructured data, like HTML. The parser makes the tokens by using lexical units like keywords and delimiters. It also ignores irrelevant information like whitespaces and comments.
After the parser has separated the data between lexical units and the irrelevant information, it discards all of the irrelevant information and passes the relevant information to the next step.
The next part of the data parsing process is syntactic analysis. This is where parse tree building happens. The parser takes the relevant tokens from the lexical analysis step and arranges them into a tree. Any further irrelevant tokens, like semicolons and curly braces, are added to the nesting structure of the tree.
Once the parse tree is finished, then you're left with relevant information in a structured format that can be saved in any file type. There are several different ways to build a data parser, from creating one programmatically to using existing tools. It depends on your business needs, how much time you have, what your budget is, and a few other factors.
To get started, let's take a look at HTML parsing libraries.
HTML parsing libraries
HTML parsing libraries are great for adding automation to your web scraping flow. You can connect many of these libraries to your web scraper via API calls and parse data as you receive it.
Here are a few popular HTML parsing libraries:
Scrapy or BeautifulSoup
These are libraries written in Python. BeautifulSoup is a Python library for pulling data out of HTML and XML files. Scrapy is a data parser that can also be used for web scraping. When it comes to web scraping with Python, there are a lot of options available and it depends on how hands-on you want to be.
Cheerio
If you're used to working with Javascript, Cheerio is a good option. It parses markup and provides an API for manipulating the resulting data structure. You could also use Puppeteer. This can be used to generate screenshots and PDFs of specific pages that can be saved and further parsed with other tools. There are many other JavaScript-based web scrapers and web parsers.
JSoup
For those that work primarily with Java, there are options for you as well. JSoup is one option. It allows you to work with real-world HTML through its API for fetching URLs and extracting and manipulating data. It acts as both a web scraper and a web parser. It can be challenging to find other Java options that are open-source, but it's definitely worth a look.
Nokogiri
There's an option for Ruby as well. Take a look at Nokogiri. It allows you to work with HTML and HTML with Ruby. It has an API similar to the other packages in other languages that lets you query the data you've retrieved from web scraping. It adds an extra layer of security because it treats all documents as untrusted by default. Data parsing in Ruby can be tricky as it can be harder to find gems you can work with.
Regular expression
Now that you have an idea of what libraries are available for your web scraping and data parsing needs, let's address a common issue with HTML parsing, regular expressions. Sometimes data isn't well-formatted inside of an HTML tag and we need to use regular expressions to extract the data we need.
You can build regular expressions to get exactly what you need from difficult data. Tools like regex101 can be an easy way to test out whether you're targeting the correct data or not. For example, you might want to get your data specifically from all of the paragraph tags on a web page. That regular expression might look something like this:
/<p>(.*)<\/p>/
The syntax for regular expressions changes slightly depending on which programming language you're working with. Most of the time, if you're working with one of the libraries we listed above or something similar, you won't have to worry about generating regular expressions.
If you aren't interested in using one of those libraries, you might consider building your own parser. This can be challenging, but potentially worth the effort if you're working with extremely complex data structures.
Building your own parser
When you need full control over how your data is parsed, building your own tool can be a powerful option. Here are a few things to consider before building your own parser.
A custom parser can be written in any programming language you like. You can make it compatible with other tools you're using, like a web crawler or web scraper, without worrying about integration issues.
In some cases, it might be cost-effective to build your own tool. If you already have a team of developers in-house, it might not too big of a task for them to accomplish.
You have granular control over everything. If you want to target specific tags or keywords, you can do that. Any time you have an update to your strategy, you won't have many problems with updating your data parser.
Although on the other hand, there are a few challenges that come with building your own parser.
The HTML of pages is constantly changing. This could become a maintenance issue for your developers. Unless you foresee your parsing tool becoming of huge importance to your business, taking that time from product development might not be effective.
It can be costly to build and maintain your own data parser. If you don't have a developer team, contracting the work is an option but that could lead to step bills based on developers' hourly rates. There's also the cost of ramping up developers that are new to the project as they figure out how things work.
You will also need to buy, build, and maintain a server to host your custom parser on. It has to be fast enough to handle all of the data that you send through it or else you might run into issues with parsing data consistently. You'll also have to make sure that server stays secure since you might be parsing sensitive data.
Having this level of control can be nice if data parsing is a big part of your business, otherwise, it could add more complexity than is necessary. There are plenty of reasons for wanting a custom parser, just make sure that it's worth the investment over using an existing tool.
Parsing schema.org meta data
There's also another way to parse web data through a website's schema. Web schema standards are managed by schema.org, a community that promotes schema for structured data on the web. Web schema is used to help search engines understand information on web pages and provide better results.
There are many practical reasons people want to parse schema metadata. For example, companies might want to parse schema for an e-commerce product to find updated prices or descriptions. Journalists could parse certain web pages to get information for their news articles. There are also website that might aggregate data like recipes, how-to guides, and technical articles.
Schema comes in different formats. You'll hear about JSON-LD, RDFa, and Microdata schema. These are the formats you'll likely be parsing.
JSON-LD is JavaScript Object Notation for Linked Data. This is made of multi-dimensional arrays. It's implemented using the schema.org standards in terms of SEO. JSON-LD is generally more simple to implement because you can paste the markup directly in an HTML document.
RDFa (Resource Description Framework in Attributes) is recommended by the World Wide Web Consortium (W3C). It's used to embed RDF statements in XML and HTML. One big difference between this and the other schema types is that RDFa only defines the metasyntax for semantic tagging.
Microdata is a WHATWG HTML specification that's used to nest metadata inside existing content on web pages. Microdata standards allow developers to design a custom vocabulary or use others like schema.org.
All of these schema types are easily parsable with a number of tools across different languages. There's a library from ScrapingHub, another from RDFLib.
Existing data parsing tools
We've covered a number of existing tools, but there are other great services available. For example, the ScrapingBee Google Search API. This tool allows you to scrape search results in real-time without worrying about server uptime or code maintainance. You only need an API key and a search query to start scraping and parsing web data.
There are many other web scraping tools, like JSoup, Puppeteer, Cheerio, or BeautifulSoup.
A few benefits of purchasing a web parser include:
- Using an existing tool is low maintenance.
- You don't have to invest a lot of time with development and configurations.
- You'll have access to support that's trained specifically to use and troubleshoot that particular tool.
Some of the downsides of purchasing a web parser include:
- You won't have granular control over everything the way your parser handles data. Although you will have some options to choose from.
- It could be an expensive upfront cost.
- Handling server issues will not be something you need to worry about.
Final thoughts
Parsing data is a common task handling everything from market research to gathering data for machine learning processes. Once you've collected your data using a mixture of web crawling and web scraping, it will likely be in an unstructured format. This makes it hard to get insightful meaning from it.
Using a parser will help you transform this data into any format you want whether it's JSON or CSV or any data store. You could build your own parser to morph the data into a highly specified format or you could use an existing tool to get your data quickly. Choose the option that will benefit your business the most.
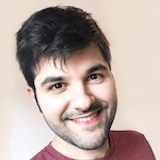
Kevin worked in the web scraping industry for 10 years before co-founding ScrapingBee. He is also the author of the Java Web Scraping Handbook.